Qt's signals and slots mechanism ensures that if you connect a signal to a slot, the slot will be called with the signal's parameters at the right time. Signals and slots can take any number of arguments of any type. They are completely type safe. Signals And Slots With Default Arguments. Signals and slots are declared at compile-time, and normally you cannot add new signals and slots to a meta-object at run-time. In some situations, it is useful to extend a meta-object while an application is running to obtain truly dynamic function invocation and introspection.
Trolltech| Documentation| Qt Quarterly |
Dynamic Signals and Slots |
by Eskil Abrahamsen Blomfeldt |
Signals and slots are declared at compile-time, and normally youcannot add new signals and slots to a meta-object at run-time. Insome situations, it is useful to extend a meta-object while anapplication is running to obtain truly dynamic functioninvocation and introspection.
The need for the dynamic addition of signals and slots arises when bindinga script interpreter, such as Qt Script for Applications (QSA), to theC++ code in your application. In QSA, it is possible to use Qt'smeta-object system to communicate between objects created in theC++ code and objects created in a script. Qt 4's meta-object systemmakes this sort of extension possible, with a little bit of hackery.
This article will get you started writing a dynamic signals and slotsbinding layer for Qt 4. We will review an abstract base class,DynamicQObject, that offers the required functionality.The 'mini-framework' presented here can serve as afoundation for doing more advanced extensions of Qt's meta-objectsystem.
Expanding the Q_OBJECT Macro |
Any QObject subclass that declares signals andslots must contain the Q_OBJECT macroin its definition. This is a magic macro that expands to a set of privatedeclarations:
Q_OBJECT is 'magic' becauseqmake and mocwill recognize it and automagically generate code for the class's meta-objectduring compilation of the project.
We want to write a DynamicQObject class that mimics part of thebehavior of the moc but that does not limit us to staticallydeclared signals and slots. Since we need to prevent the moc fromcompiling our class, we can't use theQ_OBJECT macro; instead, wewill copy its definition and insert it into our code.
The Q_OBJECT macro is defined insrc/corelib/kernel/qobjectdefs.h (which is included by<QObject>). It declares a number of functions that must bereimplemented to fully support Qt's meta-object system. For thepurposes of this article, it will be sufficient to reimplement just oneof these functions:
When a signal is emitted, Qt uses qt_metacall() to invoke theslots connected to the signal. The first parameter, call, is thenset to QMetaObject::InvokeMetaMethod.(The qt_metacall() function is also used for other types of accessto the meta-object, such as setting or getting properties.)
The second parameter is an index that uniquely identifies a signal orslot in the hierarchy of classes inherited by the current object. Thelast parameter is an array that contains pointers to arguments,preceded by a pointer to the location where the return value shouldbe placed (if any).
The DynamicQObject Class Definition |
Here's the definition of the DynamicQObject class:
The intended usage pattern of DynamicQObject involves subclassingit and adding some functionality. Whenever we add a slot to anobject, we would expect this slot to execute code when it is called.Since this part of the mechanism is dependent on the language towhich we want to bind, it must be implemented in a subclass.
The pure virtual function createSlot() returns an instance ofan appropriate subclass of the abstract class DynamicSlot thathandles function invocation for your specific language binding.DynamicSlot is defined as follows:
DynamicSlot subclasses must reimplement the call() functionto handle a slot invocation. The arguments array's first entry isa pointer to a location where we can put the return value. Thispointer is null if the signal's return type is void.
The rest of the array contains pointers to arguments passed to theslot, in order of declaration. We must cast these pointers tothe correct data types before we can use them. For example, if theslot expects a parameter of type int as its first parameter, thefollowing code would be safe:
Implementation of connectDynamicSlot() |
The connectDynamicSlot() function connects a static signaldeclared in an arbitrary QObject instance toa dynamic slot in the current DynamicQObject instance. The functiontakes a pointer to the sender object (the receiver is always this)and the signatures of the signal and slot. The function returns falseon error.
The first step is to normalize the signatures passed to the function.This will remove meaningless whitespace in the signatures, making itpossible for us to recognize them. Then, we check whether thesignatures are compatible, to avoid crashes later.
If the argument lists are compatible for the two functions, wecan start resolving the signal and slot indexes. The signal isresolved using introspection into the providedQObject object. If the signal was not found,we return false.
Resolving the (dynamic) slot is a bit more work:
We check whether the slot signature has been registered before in thesame DynamicQObject instance. If it hasn't, we add it to our listof dynamic slots.
We use aQHash<QByteArray, int>called slotIndices and aQList<DynamicSlot *> calledslotList to store the information needed for the dynamic slots.
Finally, we register the connection with Qt's meta-object system.When we notify Qt about the connection, we must add the number ofmethods inherited from QObject to our slotId.
The connectDynamicSignal() function is very similar toconnectDynamicSlot(), so we won't review it here.
Implementation of qt_metacall() |
Qt's meta-object system uses the qt_metacall() function to accessthe meta-information for a particular QObjectobject (its signals, slots, properties, etc.).
Since the call may indicate access to the meta-object of theQObject base class, we must start by callingQObject's implementation of the function.This call will return a new identifier for the slot, indexing the methods inthe upper part of the current object's class hierarchy (the current classand its subclasses).
If the QObject::qt_metacall() callreturns -1, this means that the metacall has been handled byQObject and that there is nothing to do.In that case, we return immediately. Similarly, if the metacall isn't a slotinvocation, we follow QObject's conventionand return an identifier that can be handled by a subclass.
In the case of a slot invocation, the identifier must be a valididentifier for the DynamicQObject object. We don't allowsubclasses to declare their own signals and slots.
If all goes well, we invoke the specified slot (usingDynamicSlot::call()) and return -1 to indicate that themetacall has been processed.
Implementation of emitDynamicSignal() |
The last function we need to review is emitDynamicSignal(), whichtakes a dynamic signal signature and an array of arguments. The functionnormalizes the signature then emits the signal, invoking any slotsconnected to it.
The arguments array can be assumed to contain valid pointers. A simpleexample of how to do this can be found in the accompanying example'ssource code, but as a general rule,QMetaType::construct() isyour friend when you are doing more advanced bindings.
If the (dynamic) signal exists (i.e., it has been connected to aslot), the function simply usesQMetaObject::activate() to tellQt's meta-object system that a signal is being emitted. It returnstrue if the signal has previously been connected to a slot;otherwise it returns false.
Pitfalls and Pointers |
Having explained the architecture of this simple framework, I'd liketo conclude by explaining what the framework doesn't do and howyou can expand on it or improve it to suit your needs.
- Only a subset of the features in Qt's meta-object system is supported by DynamicQObject. Ideally, we would reimplement all the functions declared by the Q_OBJECT macro.
- Declaring new signals and slots in DynamicQObject subclasses will cause an application to crash. The reason for this is that the meta-object system expects the number of methods declared in a meta-object to be static. A safer workaround would be to use a different pattern than inheritance to employ dynamic signals and slots.
- The framework is sensitive to misspellings. For instance, if you try to connect two distinct signals to the same dynamic slot and misspell the signature of the slot in one of the connect calls, the framework will create two separate slots instead of indicating a failure. To handle this in a safer way, you could implement a register() function and require the user to register each signal and slot before connecting to them.
- The framework cannot handle connections where both the signals and the slot are dynamic. This can easily be written as an extension of the DynamicQObject class.
- Removing connections is not possible with the current framework. This can easily be implemented in a similar way to establishing connections. Make sure not to change the order of entries in slotList, because the list is indexed by slot ID.
- In real-world applications, you may need to do type conversions before passing parameters between functions declared in scripts and functions declared in C++. The DynamicQObject class can be expanded to handle this in a general way by declaring a set of virtual functions that can be reimplemented for different bindings.
Copyright © 2006 Trolltech | Trademarks |
The QThread
class provides a platform-independent way to manage threads. More…
Synopsis¶
Functions¶
def
eventDispatcher
()def
exec_
()def
exit
([retcode=0])def
isFinished
()def
isInterruptionRequested
()def
isRunning
()def
loopLevel
()def
priority
()def
requestInterruption
()def
setEventDispatcher
(eventDispatcher)def
setPriority
(priority)def
setStackSize
(stackSize)def
stackSize
()def
wait
([time=ULONG_MAX])
Virtual functions¶
Slots¶
def
quit
()def
start
([priority=InheritPriority])def
terminate
()
Static functions¶
def
currentThread
()def
idealThreadCount
()def
msleep
(arg__1)def
setTerminationEnabled
([enabled=true])def
sleep
(arg__1)def
usleep
(arg__1)def
yieldCurrentThread
()
Detailed Description¶
A QThread
object manages one thread of control within the program. QThreads begin executing in run()
. By default, run()
starts the event loop by calling exec()
and runs a Qt event loop inside the thread.
You can use worker objects by moving them to the thread using moveToThread()
.
The code inside the Worker’s slot would then execute in a separate thread. However, you are free to connect the Worker’s slots to any signal, from any object, in any thread. It is safe to connect signals and slots across different threads, thanks to a mechanism called queuedconnections
.
Another way to make code run in a separate thread, is to subclass QThread
and reimplement run()
. For example:
In that example, the thread will exit after the run function has returned. There will not be any event loop running in the thread unless you call exec()
.
It is important to remember that a QThread
instance livesin
the old thread that instantiated it, not in the new thread that calls run()
. This means that all of QThread
‘s queued slots and invokedmethods
will execute in the old thread. Thus, a developer who wishes to invoke slots in the new thread must use the worker-object approach; new slots should not be implemented directly into a subclassed QThread
.
Unlike queued slots or invoked methods, methods called directly on the QThread
object will execute in the thread that calls the method. When subclassing QThread
, keep in mind that the constructor executes in the old thread while run()
executes in the new thread. If a member variable is accessed from both functions, then the variable is accessed from two different threads. Check that it is safe to do so.
Note
Care must be taken when interacting with objects across different threads. See Synchronizing Threads for details.
Managing Threads¶
QThread
will notifiy you via a signal when the thread is started()
and finished()
, or you can use isFinished()
and isRunning()
to query the state of the thread.
You can stop the thread by calling exit()
or quit()
. In extreme cases, you may want to forcibly terminate()
an executing thread. However, doing so is dangerous and discouraged. Please read the documentation for terminate()
and setTerminationEnabled()
for detailed information.
From Qt 4.8 onwards, it is possible to deallocate objects that live in a thread that has just ended, by connecting the finished()
signal to deleteLater()
.
Use wait()
to block the calling thread, until the other thread has finished execution (or until a specified time has passed).
QThread
also provides static, platform independent sleep functions: sleep()
, msleep()
, and usleep()
allow full second, millisecond, and microsecond resolution respectively. These functions were made public in Qt 5.0.
Note
wait()
and the sleep()
functions should be unnecessary in general, since Qt is an event-driven framework. Instead of wait()
, consider listening for the finished()
signal. Instead of the sleep()
functions, consider using QTimer
.
The static functions currentThreadId()
and currentThread()
return identifiers for the currently executing thread. The former returns a platform specific ID for the thread; the latter returns a QThread
pointer.
To choose the name that your thread will be given (as identified by the command ps-L
on Linux, for example), you can call setObjectName()
before starting the thread. If you don’t call setObjectName()
, the name given to your thread will be the class name of the runtime type of your thread object (for example, 'RenderThread'
in the case of the Mandelbrot Example , as that is the name of the QThread
subclass). Note that this is currently not available with release builds on Windows.
See also
QThreadStorage
Mandelbrot ExampleSemaphores ExampleWait Conditions Example
QThread
([parent=None])¶Constructs a new QThread
to manage a new thread. The parent
takes ownership of the QThread
. The thread does not begin executing until start()
is called.
See also
PySide2.QtCore.QThread.
Priority
¶This enum type indicates how the operating system should schedule newly created threads.
Constant | Description |
---|---|
QThread.IdlePriority | scheduled only when no other threads are running. |
QThread.LowestPriority | scheduled less often than . |
QThread.LowPriority | scheduled less often than . |
QThread.NormalPriority | the default priority of the operating system. |
QThread.HighPriority | scheduled more often than . |
QThread.HighestPriority | scheduled more often than . |
QThread.TimeCriticalPriority | scheduled as often as possible. |
QThread.InheritPriority | use the same priority as the creating thread. This is the default. |
PySide2.QtCore.QThread.
currentThread
()¶- Return type
Returns a pointer to a QThread
which manages the currently executing thread.
PySide2.QtCore.QThread.
eventDispatcher
()¶- Return type
Returns a pointer to the event dispatcher object for the thread. If no event dispatcher exists for the thread, this function returns None
.
PySide2.QtCore.QThread.
exec_
()¶int
Enters the event loop and waits until exit()
is called, returning the value that was passed to exit()
. The value returned is 0 if exit()
is called via quit()
.
This function is meant to be called from within run()
. It is necessary to call this function to start event handling.
See also
PySide2.QtCore.QThread.
exit
([retcode=0])¶retcode – int
Tells the thread’s event loop to exit with a return code.
After calling this function, the thread leaves the event loop and returns from the call to exec()
. The exec()
function returns returnCode
.
By convention, a returnCode
of 0 means success, any non-zero value indicates an error.
Note that unlike the C library function of the same name, this function does return to the caller – it is event processing that stops.
No QEventLoops will be started anymore in this thread until exec()
has been called again. If the eventloop in exec()
is not running then the next call to exec()
will also return immediately.
PySide2.QtCore.QThread.
idealThreadCount
()¶int
Returns the ideal number of threads that can be run on the system. This is done querying the number of processor cores, both real and logical, in the system. This function returns 1 if the number of processor cores could not be detected.
PySide2.QtCore.QThread.
isFinished
()¶bool
Returns true
if the thread is finished; otherwise returns false
.
See also
PySide2.QtCore.QThread.
isInterruptionRequested
()¶bool
Return true if the task running on this thread should be stopped. An interruption can be requested by requestInterruption()
.
This function can be used to make long running tasks cleanly interruptible. Never checking or acting on the value returned by this function is safe, however it is advisable do so regularly in long running functions. Take care not to call it too often, to keep the overhead low.
See also
PySide2.QtCore.QThread.
isRunning
()¶Qt Signal Slot Default Parameter
bool
Returns true
if the thread is running; otherwise returns false
.
PySide2.QtCore.QThread.
loopLevel
()¶int
Returns the current event loop level for the thread.
Note
This can only be called within the thread itself, i.e. when it is the current thread.
PySide2.QtCore.QThread.
msleep
(arg__1)¶arg__1 – long
Forces the current thread to sleep for msecs
milliseconds.
Avoid using this function if you need to wait for a given condition to change. Instead, connect a slot to the signal that indicates the change or use an event handler (see event()
).
Note
This function does not guarantee accuracy. The application may sleep longer than msecs
under heavy load conditions. Some OSes might round msecs
up to 10 ms or 15 ms.
PySide2.QtCore.QThread.
priority
()¶- Return type
Returns the priority for a running thread. If the thread is not running, this function returns InheritPriority
.
See also
Priority
setPriority()
start()
PySide2.QtCore.QThread.
quit
()¶Tells the thread’s event loop to exit with return code 0 (success). Equivalent to calling exit
(0).
This function does nothing if the thread does not have an event loop.
PySide2.QtCore.QThread.
requestInterruption
()¶Request the interruption of the thread. That request is advisory and it is up to code running on the thread to decide if and how it should act upon such request. This function does not stop any event loop running on the thread and does not terminate it in any way.
See also
PySide2.QtCore.QThread.
run
()¶The starting point for the thread. After calling start()
, the newly created thread calls this function. The default implementation simply calls exec()
.
You can reimplement this function to facilitate advanced thread management. Returning from this method will end the execution of the thread.
PySide2.QtCore.QThread.
setEventDispatcher
(eventDispatcher)¶eventDispatcher – QAbstractEventDispatcher
Sets the event dispatcher for the thread to eventDispatcher
. This is only possible as long as there is no event dispatcher installed for the thread yet. That is, before the thread has been started with start()
or, in case of the main thread, before QCoreApplication
has been instantiated. This method takes ownership of the object.
See also
PySide2.QtCore.QThread.
setPriority
(priority)¶priority – Priority
This function sets the priority
for a running thread. If the thread is not running, this function does nothing and returns immediately. Use start()
to start a thread with a specific priority.
The priority
argument can be any value in the QThread::Priority
enum except for InheritPriorty
.
The effect of the priority
parameter is dependent on the operating system’s scheduling policy. In particular, the priority
will be ignored on systems that do not support thread priorities (such as on Linux, see http://linux.die.net/man/2/sched_setscheduler for more details).
PySide2.QtCore.QThread.
setStackSize
(stackSize)¶stackSize – uint
Sets the maximum stack size for the thread to stackSize
. If stackSize
is greater than zero, the maximum stack size is set to stackSize
bytes, otherwise the maximum stack size is automatically determined by the operating system.
Warning
Most operating systems place minimum and maximum limits on thread stack sizes. The thread will fail to start if the stack size is outside these limits.
PySide2.QtCore.QThread.
setTerminationEnabled
([enabled=true])¶enabled – bool
Enables or disables termination of the current thread based on the enabled
parameter. The thread must have been started by QThread
.
When enabled
is false, termination is disabled. Future calls to terminate()
will return immediately without effect. Instead, the termination is deferred until termination is enabled.
Qt Slot With Default Parameters
When enabled
is true, termination is enabled. Future calls to terminate()
will terminate the thread normally. If termination has been deferred (i.e. terminate()
was called with termination disabled), this function will terminate the calling thread immediately . Note that this function will not return in this case.
See also
PySide2.QtCore.QThread.
sleep
(arg__1)¶arg__1 – long
Forces the current thread to sleep for secs
seconds.
Avoid using this function if you need to wait for a given condition to change. Instead, connect a slot to the signal that indicates the change or use an event handler (see event()
).
Note
This function does not guarantee accuracy. The application may sleep longer than secs
under heavy load conditions.
PySide2.QtCore.QThread.
stackSize
()¶uint
Returns the maximum stack size for the thread (if set with setStackSize()
); otherwise returns zero.
See also
PySide2.QtCore.QThread.
start
([priority=InheritPriority])¶priority – Priority
Begins execution of the thread by calling run()
. The operating system will schedule the thread according to the priority
parameter. If the thread is already running, this function does nothing.
The effect of the priority
parameter is dependent on the operating system’s scheduling policy. In particular, the priority
will be ignored on systems that do not support thread priorities (such as on Linux, see the sched_setscheduler documentation for more details).
PySide2.QtCore.QThread.
terminate
()¶Terminates the execution of the thread. The thread may or may not be terminated immediately, depending on the operating system’s scheduling policies. Use wait()
after , to be sure.
When the thread is terminated, all threads waiting for the thread to finish will be woken up.
Warning
This function is dangerous and its use is discouraged. The thread can be terminated at any point in its code path. Threads can be terminated while modifying data. There is no chance for the thread to clean up after itself, unlock any held mutexes, etc. In short, use this function only if absolutely necessary.
Termination can be explicitly enabled or disabled by calling setTerminationEnabled()
. Calling this function while termination is disabled results in the termination being deferred, until termination is re-enabled. See the documentation of setTerminationEnabled()
for more information.
PySide2.QtCore.QThread.
usleep
(arg__1)¶arg__1 – long
Forces the current thread to sleep for usecs
microseconds.
Avoid using this function if you need to wait for a given condition to change. Instead, connect a slot to the signal that indicates the change or use an event handler (see event()
).
Note
This function does not guarantee accuracy. The application may sleep longer than usecs
under heavy load conditions. Some OSes might round usecs
up to 10 ms or 15 ms; on Windows, it will be rounded up to a multiple of 1 ms.
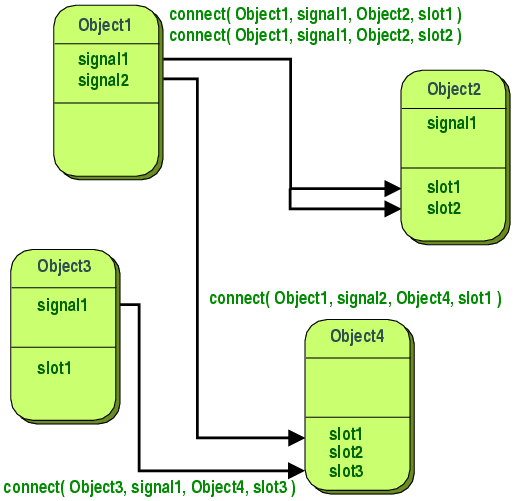
PySide2.QtCore.QThread.
wait
([time=ULONG_MAX])¶time – long
Qt Slot With Default Arguments
bool
Blocks the thread until either of these conditions is met:
The thread associated with this
QThread
object has finished execution (i.e. when it returns fromrun()
). This function will return true if the thread has finished. It also returns true if the thread has not been started yet.time
milliseconds has elapsed. Iftime
is ULONG_MAX (the default), then the wait will never timeout (the thread must return fromrun()
). This function will return false if the wait timed out.
This provides similar functionality to the POSIX pthread_join()
function.
See also
PySide2.QtCore.QThread.
yieldCurrentThread
()¶Yields execution of the current thread to another runnable thread, if any. Note that the operating system decides to which thread to switch.
© 2018 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.